jzmn.setPhotoset
is a javascript function for beautifully resizing images in a photoset, without cropping or aspect-ratio distortion, such that all images in a row are of the same height.jzmn.setPhotoset
makes use of percentage widths, so that the photoset can work for all sizes, and can be resized without problem.
Basic Usage
The general format of setPhotoset
is shown below:
setPhotoset(element,userOptions,grouping);
element
refers to the element node, nodelist of elements, or CSS-selector string of the photosets you want to set.
userOptions
is an optional object containing customizable options for the function (listed below).
grouping
is an optional CSS-selector-friendly string meant to group photosets together in a class for similar styling. If not set, grouping
will be randomly generated.
User Options
Options | Type | Default | Description |
---|---|---|---|
layout | String || [Number] | null | defines layout of photoset (number of items per row. e.g. '121' or [1,2,1]). If falsy, layout information is aquired from the attribute name defined by layoutAttribute |
layoutAttribute | String | data-layout | attribute name from which layout information will be derived, if layout property is falsy. |
immediate | Boolean | false | If false, waits for all children defined by childItem to load before executing layout application. If true immediately applies layout, making use of height and width data provided in the attributes defined in childHeight and childWidth of childItems. |
childItem | String | img | CSS-selector string that determines which children inside element is used to create grid. |
childHeight | String | height | name of attribute on each childItem that lists item's height. |
childWidth | String | width | name of attribute on each childItem that lists item's width. |
gutter | Number || String | 0 | value that sets spacing between items. If string, must be usable inside calc(). If a number, is set in px. |
createSheet | Boolean | true | boolean determining whether a stylesheet is to be created containing the styling required for layouting (if it doesn't yet exist). Set to false if you wish to supply your own stylesheet. See setphotoset-example.css for sample. |
Examples
Example 1: Basic Usage
HTML
<div class="photoset" id="#photoset1">
<img src="1.png" />
<img src="2.png" />
<img src="3.png" />
<img src="4.png" />
<img src="5.png" />
</div>
Javascript
var photoset = document.querySelector("#photoset1");
setPhotoset(photoset,{layout:"23", gutter:"5px"});
Result

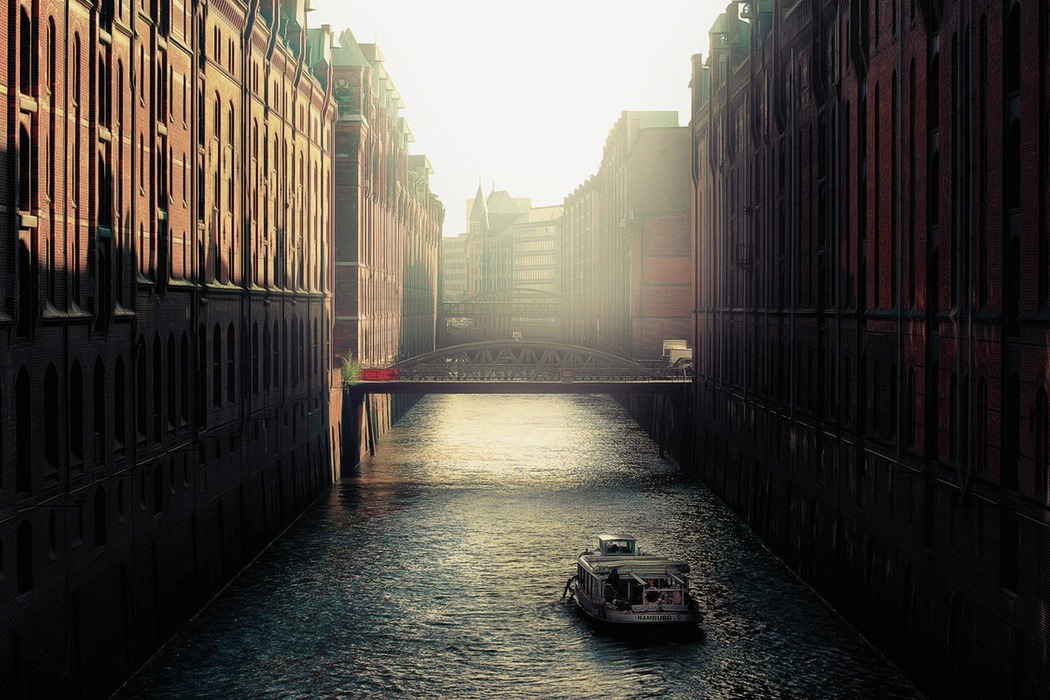

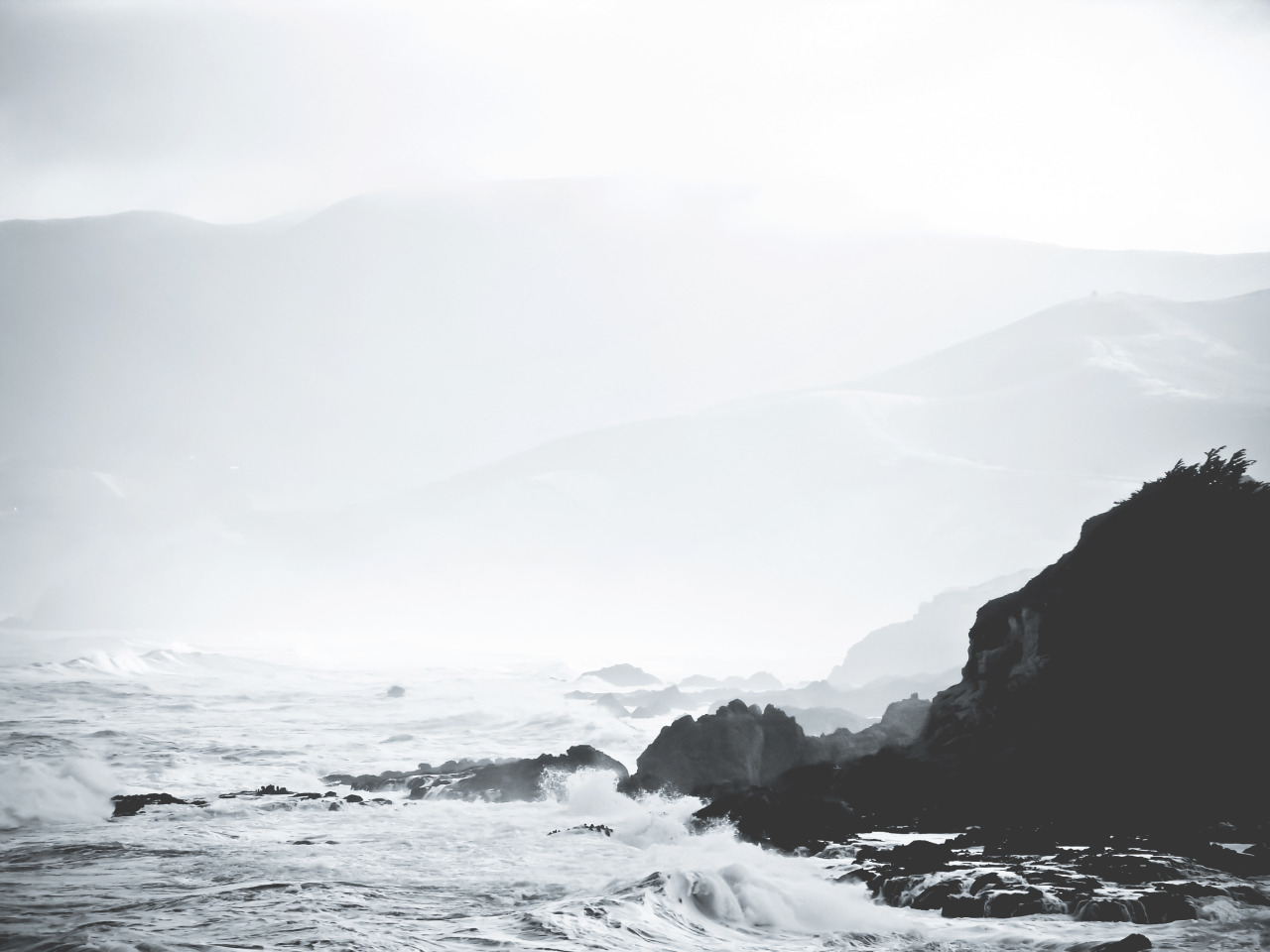

Example 2: Multiple Photosets
By defining a layoutAttribute
property and then setting it as an attribute on each individual photoset (or setting a 'data-layout' attribute which is the default value for the option), multiple sets can be targeted and styled by the same line of javascript while applying different layouts.
HTML
<div class="photoset" id="#photoset2-1" data-layout="[2,3]">
<img src="1.png" />
<img src="2.png" />
<img src="3.png" />
<img src="4.png" />
<img src="5.png" />
</div>
<div class="photoset" id="#photoset2-2" data-layout="121" >
<img src="6.png" />
<img src="7.png" />
<img src="8.png" />
<img src="9.png" />
</div>
Javascript
var photosets = document.querySelectorAll(".photoset"); setPhotoset(photosets, {gutter: 5});
Result

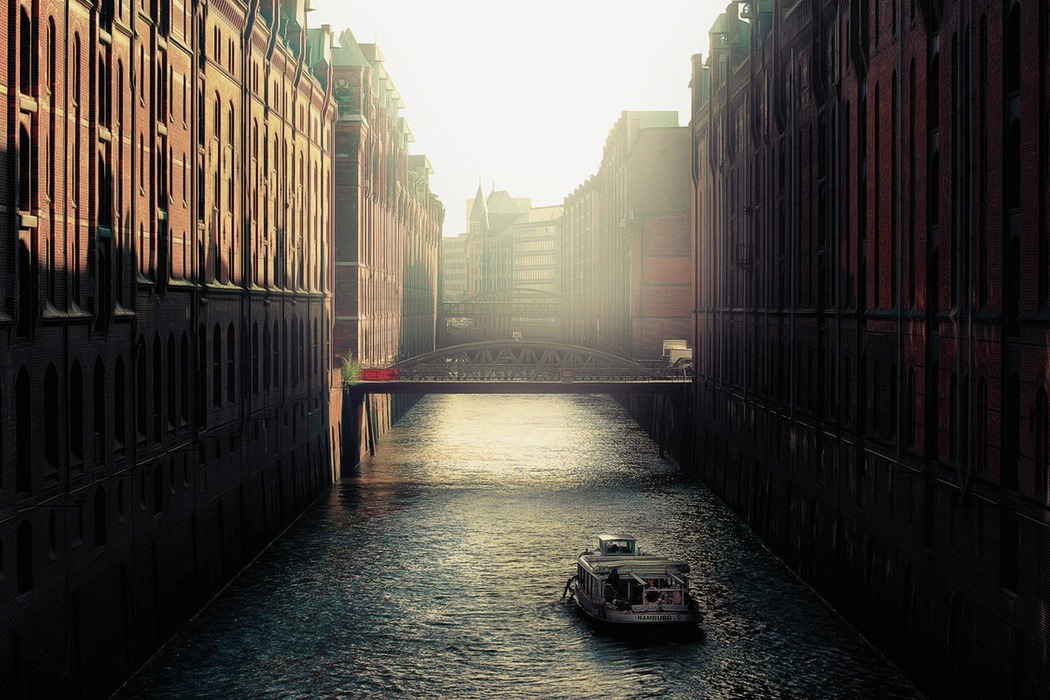

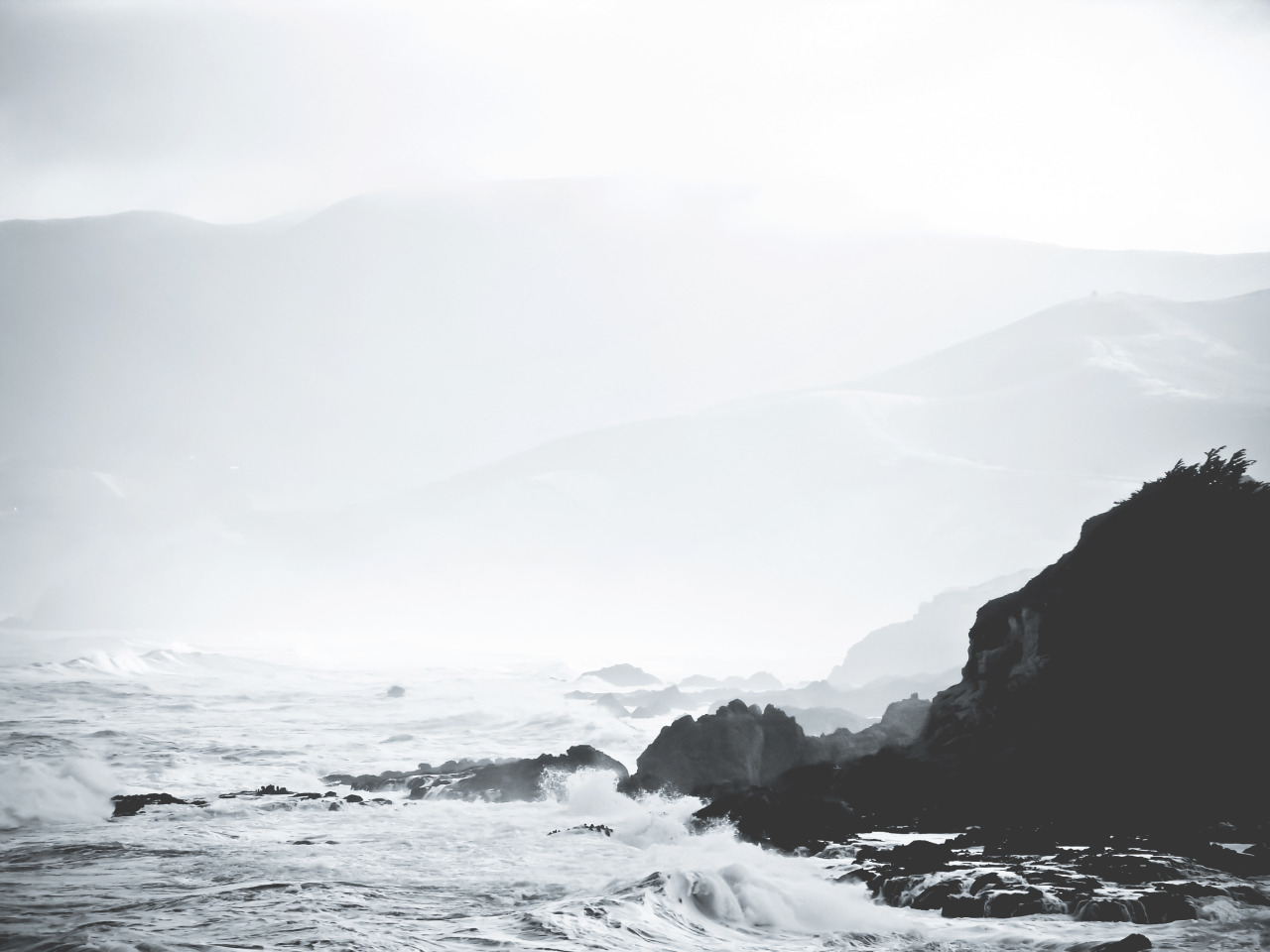




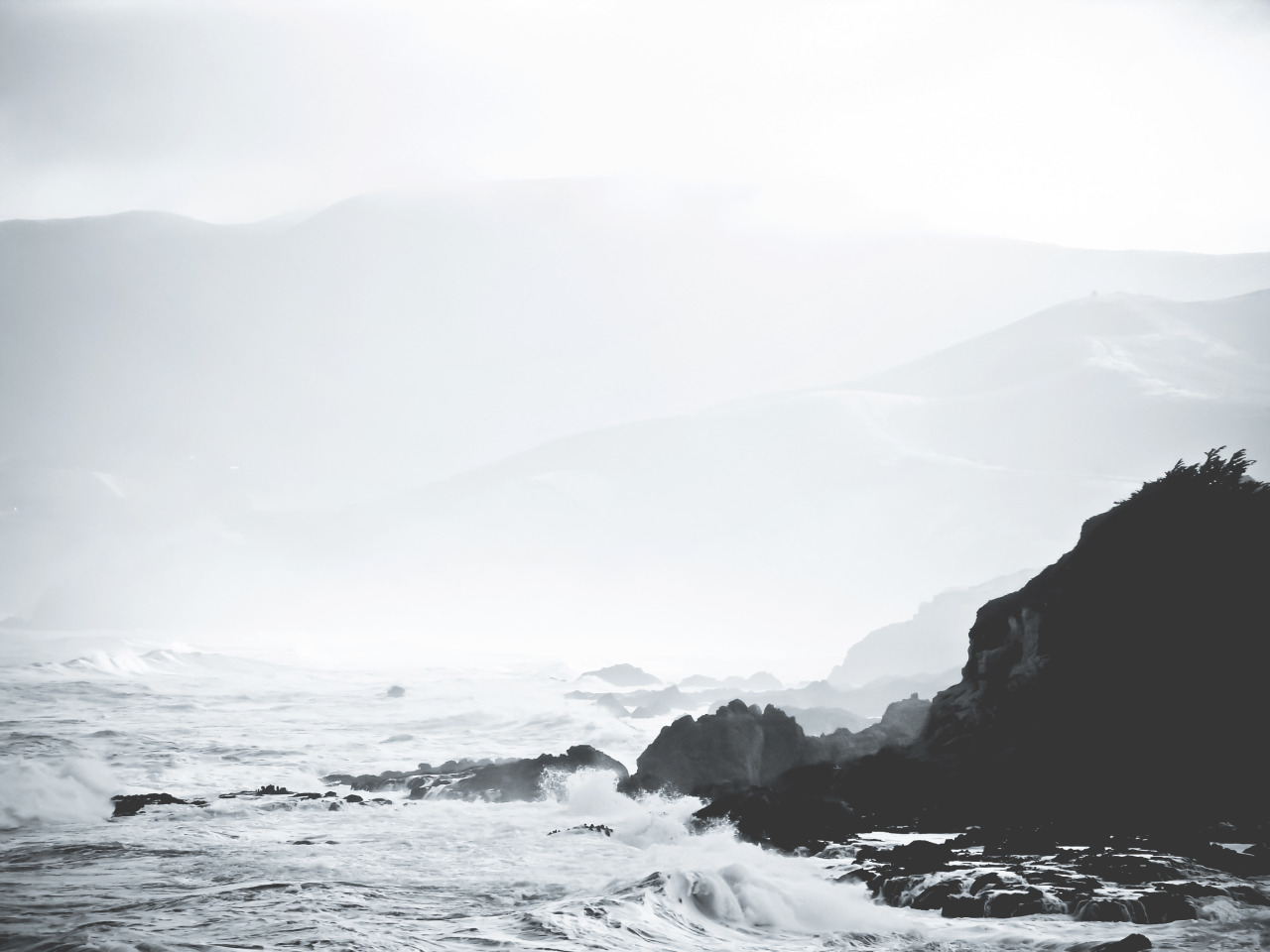
Example 3: Setting Immediately
If the height and width information is already available (e.g. if the 'height' and 'width' attributes on the image are present), then you can set immediate
to true and the layout will be calculated and set without needing to wait for the images to load.
HTML
<div class="photoset" id="#photoset3" data-layout="12" >
<a href="1.png">
<img src="1.png" height="2448" width"3264" />
</a>
<a href="2.png" >
<img src="2.png" height="700" width"1050" />
</a>
<a href="1.png" >
<img src="1.png" height="1575" width"1050" />
</a>
</div>
Javascript
var photoset = document.querySelector("#photoset3");
setPhotoset(photoset,{
immediate: true,
gutter: "0.25em"
});